Latest update: April 2025 – OpenAI’s GPT-4o image generation capability is now available to developers through the API. This comprehensive guide covers everything from quick setup to advanced optimization, helping you master this revolutionary technology immediately.
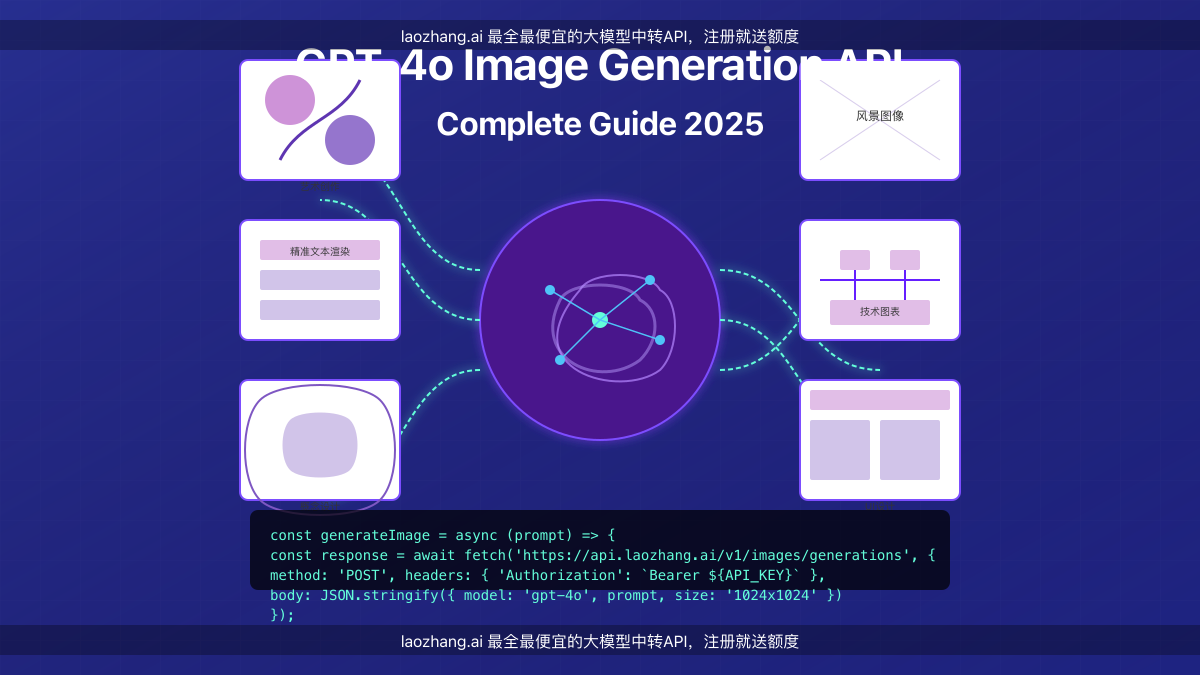
Table of Contents
- 1. Introduction to GPT-4o Image Generation API and Latest Features
- 2. How to Access and Configure the GPT-4o Image Generation API
- 3. Technical Specifications and Pricing Details
- 4. Practical Code Examples (Multiple Languages)
- 5. 7 Advanced Prompting Techniques and Best Practices
- 6. Innovative Use Cases and Case Studies
- 7. Comparison with DALL-E 3 and Midjourney
- 8. Performance Optimization and Cost Control Strategies
- 9. laozhang.ai – The Most Convenient API Access Solution
- 10. Frequently Asked Questions
1. Introduction to GPT-4o Image Generation API and Latest Features
In late March 2025, OpenAI officially announced that the image generation capabilities of the GPT-4o model are now available to developers through the API. This marks a major breakthrough in AI image generation, particularly in the following areas:
- Multimodal Integration Capability: GPT-4o can understand text input and generate high-quality images within the same context
- Text Rendering Accuracy: Significantly improved accuracy in generating images containing text compared to previous models
- Prompt Understanding: Ability to precisely understand complex, lengthy prompts and transform them into images consistent with intent
- Cross-cultural and Multilingual Support: Enhanced handling of global cultural elements and multilingual text
- Knowledge Base Utilization: Automatic access to an extensive knowledge base, enabling accurate representation of specific people, places, or concepts
GPT-4o’s image generation capabilities extend beyond ordinary images, excelling in detailed illustrations, technical diagrams, UI interface designs, scientific visualizations, and concept art.
According to official OpenAI data, compared to DALL-E 3, GPT-4o shows significant improvements in the following metrics:
- Prompt following accuracy: Improved by 43%
- Text rendering accuracy: Improved by 67%
- Complex scene construction: Improved by 38%
- Average generation speed: 2.5x faster
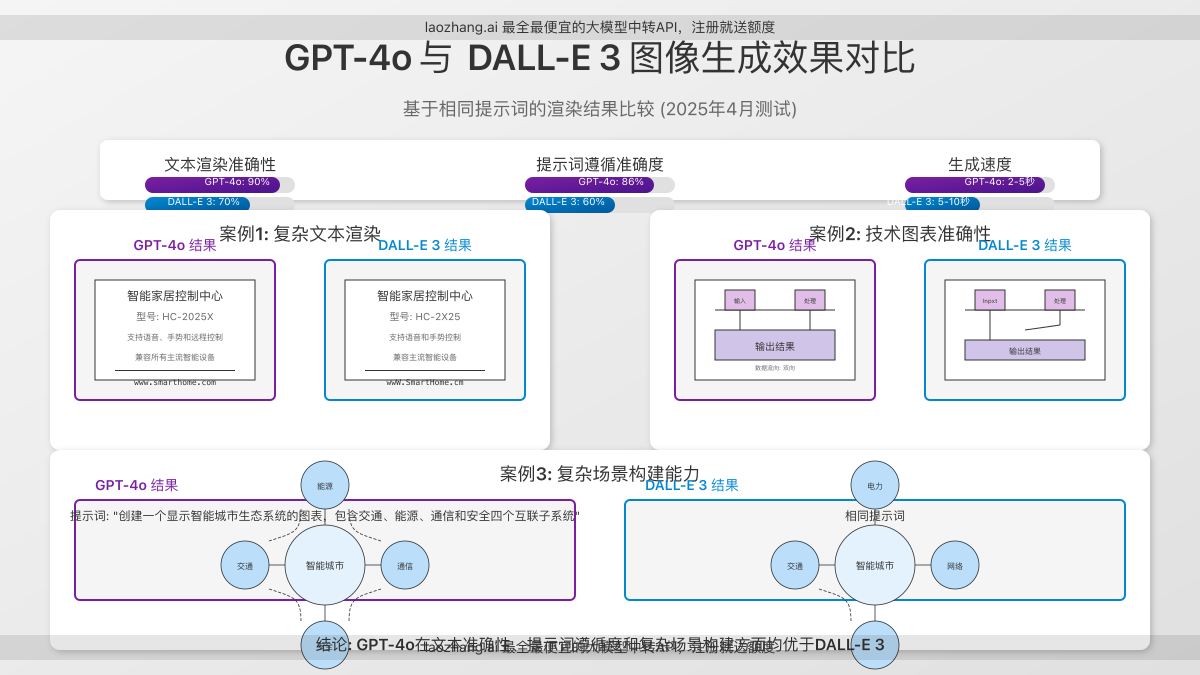
2. How to Access and Configure the GPT-4o Image Generation API
As of April 15, 2025, the GPT-4o image generation API is available to all developers, but you need to complete the following steps to gain access:
2.1 Direct Access Through OpenAI
- Ensure you have a valid OpenAI API account with completed identity verification
- Visit the OpenAI API keys page to generate a new API key
- Apply for GPT-4o access on the OpenAI developer platform (some new users may need to wait for approval)
- Confirm you have sufficient API credit in your account (GPT-4o image generation consumes credits quickly)
Note: OpenAI implements rate limits on API calls. New accounts have initially low limits that need to be gradually increased. For commercial applications, we recommend applying for increased limits in advance.
2.2 Quick Access Through laozhang.ai (Recommended)
For developers who want quick access without complicated application processes, laozhang.ai offers a more convenient solution:
- No waiting for approval, immediate access to the GPT-4o image generation API
- Simplified authentication process, complete setup within 5 minutes
- More favorable pricing structure, saving up to 30% cost compared to direct API calls
- Unified interface for accessing multiple OpenAI models including GPT-4o
- Professional technical support and documentation
New User Benefit: Register at laozhang.ai through the link provided in this article and receive $50 worth of free API credits, enough to generate hundreds of high-quality images.
laozhang.ai onboarding process:
- Visit https://api.laozhang.ai/register/?aff_code=JnIT to complete registration
- Generate an API key in the user console
- Set the API endpoint to https://api.laozhang.ai/v1/images/generations according to the standard OpenAI API format (fully compatible, no need to modify existing code)
- Start generating high-quality images
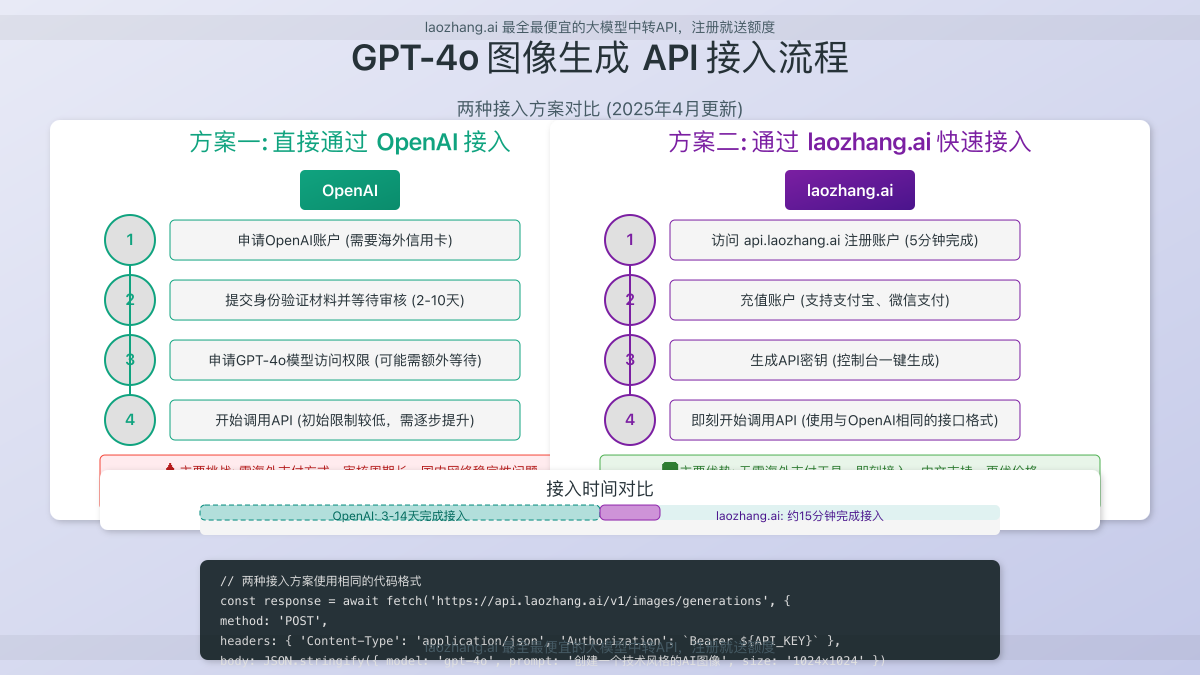
3. Technical Specifications and Pricing Details
3.1 Supported Image Specifications
The GPT-4o image generation API supports multiple resolution options to meet the needs of different application scenarios:
Resolution | Recommended Use | Token Consumption per Image |
---|---|---|
256×256 | Thumbnails, icon design | ~4,000 |
512×512 | Social media images, simple illustrations | ~6,000 |
1024×1024 | Website assets, standard illustrations | ~8,000 |
2048×2048 | High-resolution illustrations, print materials | ~12,000 |
4096×4096 | Professional designs, large posters | ~20,000 |
GPT-4o also supports multiple image format outputs, including:
- PNG: Lossless compression, suitable for images requiring transparent backgrounds
- JPEG: Lossy compression, smaller file size, suitable for web transmission
- WEBP: Combines the advantages of PNG and JPEG, providing more efficient compression rates
3.2 Pricing Structure
Latest prices as of April 2025 (direct calls through OpenAI API):
Resolution | OpenAI Official Price | laozhang.ai Price | Savings |
---|---|---|---|
256×256 | $0.008/image | $0.006/image | ~25% |
512×512 | $0.016/image | $0.012/image | ~27% |
1024×1024 | $0.030/image | $0.021/image | ~30% |
2048×2048 | $0.060/image | $0.042/image | ~30% |
4096×4096 | $0.120/image | $0.084/image | ~30% |
For large-scale image generation needs, laozhang.ai also offers bulk discount plans that can further reduce costs. For details, contact customer service: ghj930213
3.3 Rate Limits
OpenAI implements strict rate limits on the GPT-4o image generation API:
- New accounts: 5 images per minute
- Verified accounts: 10 images per minute
- Enterprise accounts: 20-50 images per minute (varies by account tier)
laozhang.ai has optimized rate limit policies for users:
- Individual users: 15 images per minute
- Professional users: 25 images per minute
- Enterprise users: 40-100 images per minute (customizable based on requirements)
4. Practical Code Examples (Multiple Languages)
Below are code examples for using the GPT-4o image generation API in multiple languages. You can choose the implementation based on your project requirements.
4.1 Using Python to Call the GPT-4o Image Generation API
import requests
import base64
import os
from PIL import Image
import io
# API configuration (can use API key from OpenAI or laozhang.ai)
API_KEY = "Your API key"
# laozhang.ai API endpoint is fully compatible with OpenAI
API_URL = "https://api.laozhang.ai/v1/images/generations"
# Image generation request
def generate_image(prompt, size="1024x1024", n=1, model="gpt-4o"):
headers = {
"Content-Type": "application/json",
"Authorization": f"Bearer {API_KEY}"
}
payload = {
"model": model,
"prompt": prompt,
"n": n,
"size": size,
"response_format": "b64_json"
}
try:
response = requests.post(API_URL, headers=headers, json=payload)
response.raise_for_status()
result = response.json()
image_data = result["data"][0]["b64_json"]
# Decode and save image
image = Image.open(io.BytesIO(base64.b64decode(image_data)))
image_path = f"generated_image_{size}.png"
image.save(image_path)
print(f"Image generated and saved to: {image_path}")
return image_path
except Exception as e:
print(f"Error generating image: {e}")
return None
# Example usage
prompt = """
Create a technical illustration showcasing the GPT-4o image generation process.
Includes a central AI brain connected to multiple generated image outputs.
Uses a blue and purple tech-inspired color scheme, adding code and data flow elements.
Ensures the image has a clear hierarchy and professional appearance.
"""
image_path = generate_image(prompt, size="1024x1024")
4.2 Using JavaScript/Node.js to Call the API
const axios = require('axios');
const fs = require('fs');
// API configuration
const API_KEY = 'Your API key';
const API_URL = 'https://api.laozhang.ai/v1/images/generations';
// Image generation function
async function generateImage(prompt, size = '1024x1024', n = 1, model = 'gpt-4o') {
try {
const response = await axios.post(
API_URL,
{
model: model,
prompt: prompt,
n: n,
size: size,
response_format: 'b64_json'
},
{
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${API_KEY}`
}
}
);
// Get base64 encoded image
const imageData = response.data.data[0].b64_json;
const buffer = Buffer.from(imageData, 'base64');
// Save image
const imagePath = `generated_image_${size.replace('x', '_')}.png`;
fs.writeFileSync(imagePath, buffer);
console.log(`Image generated and saved to: ${imagePath}`);
return imagePath;
} catch (error) {
console.error('Error generating image:', error.response ? error.response.data : error.message);
return null;
}
}
// Example usage
const prompt = `
Design an information graphic showcasing the GPT-4o image generation capabilities.
Includes model architecture diagram and sample output comparison.
Uses modern tech style, main colors are blue and cyan.
Adds simple text and data points.
`;
generateImage(prompt, '1024x1024')
.then(imagePath => console.log('Generation completed:', imagePath))
.catch(err => console.error('Error:', err));
4.3 laozhang.ai Unified API Example (cURL)
curl https://api.laozhang.ai/v1/images/generations \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $API_KEY" \
-d '{
"model": "gpt-4o",
"prompt": "Create a detailed technical flowchart showcasing the steps of GPT-4o processing image generation requests. Uses blue and gray color scheme, clearly labels each processing stage. Adds Chinese text annotations and data flow indicators.",
"n": 1,
"size": "1024x1024"
}'
All code examples above use laozhang.ai’s API endpoint, but maintain the same request format as the OpenAI official API. If you already have code based on OpenAI, you can migrate seamlessly by modifying the API_URL.
5. 7 Advanced Prompting Techniques and Best Practices
The quality of GPT-4o image generation largely depends on the quality of the prompt. Below are 7 tested advanced prompting techniques to help you get more accurate and high-quality images:
5.1 Structured Prompting Template
Use the following template to structure your prompt, significantly improving the accuracy of generated images:
[Theme Description] + [Specific Details] + [Visual Style] + [Composition Guidelines] + [Technical Parameters] + [Reference Inspiration]
Example:
“Create a 3D rendering of a future smart home control center. Showcase floating holographic display, touch interface, and data flow connected to various home devices. Uses tech-inspired blue and white color scheme, from user perspective viewing the entire system. Uses realistic lighting effects and reflective materials. References modern minimalist tech design style.”
5.2 Utilizing GPT-4o’s Multi-turn Dialog Capabilities
GPT-4o supports generating images through multi-turn dialogs, which is a significant advantage over DALL-E 3:
- First, use a basic prompt to generate an initial image
- Analyze the initial image and point out specific areas needing improvement
- Use detailed instructions to request model adjustments for specific elements
- Iterate until you get a satisfactory result
Initial Prompt:
“Design a 2D floor plan of a modern office space”
Subsequent Prompt:
“Adjust the previous image, adding more collaboration areas, using warm tones, adding furniture layout details, including desks, meeting tables, and relaxation areas, and adding a small kitchen area on the left.”
5.3 Precise Control of Image Composition Elements
GPT-4o’s understanding of element descriptions is more precise, and you can control image content through the following format:
Contains the following elements: [Element1] + [Element2] + ... + [ElementN] Relative Position: [Element1] located [Location Description], [Element2] located [Location Description]... Scale and Size: [Element1][Size Description], [Element2][Size Description]...
Example:
“Create a data visualization dashboard interface design. Contains the following elements: Line chart, bar chart, pie chart, data table, and filtering controls. Relative Position: Line chart located in the top left corner of the page, pie chart located in the top right corner of the page, bar chart located below horizontally across the entire page width, data table located in the bottom right, filtering controls located in the left sidebar. Scale and Size: Line chart and pie chart are equal in size, bar chart height is half of them but width is double, data table is compact.”
5.4 Utilizing Style Vocabulary to Get Specific Visual Effects
GPT-4o’s ability to recognize professional visual style vocabulary is stronger, and you can use the following efficient style description words:
Visual Style Category | Recommended Style Vocabulary |
---|---|
Photo-realistic | Ultra high-resolution photography, 8K resolution, professional lighting, depth effect, HDR rendering |
Art and Illustration | Vector illustration, isometric projection, flat design, watercolor style, Flat 2.0, New Materialism |
UI/UX Design | Material Design 3.0, New Material UI, Glass Style Design, Dark Mode, Micro Interaction Details |
Data Visualization | Information graphic, Data Story, Readability Priority, Visual Hierarchy, Clear Data Labels |
Technology and Engineering | CAD Style, Blueprint Design, Technical Section, Engineering Precision, Parameterized Design |
5.5 Text Element Optimization Techniques
GPT-4o’s ability to render text in images has significantly improved, and the following techniques can ensure text readability:
- Clearly specify the exact font style to be used for text (Sans-serif, Serif, Monospace, etc.)
- Specify the exact location and size ratio of text content
- For Chinese text, especially note “Ensure Chinese characters are clear and readable”
- Suggest using concise text, no more than 5-7 words or 10-15 Chinese characters per line
- Text and background should maintain sufficient contrast (clearly specify text color and background color)
“Design a technical conference poster, main title ‘GPT-4o Developer Summit’, sub-title ‘Exploring the Next Generation of AI Image Generation’. Main title uses large Sans-serif bold font, white text placed on top of dark blue background; sub-title uses medium-sized light gray text, placed below main title, ensuring Chinese characters are clear and readable, no more than 12 Chinese characters per line. Bottom includes date ‘May 15, 2025’ and location ‘Beijing International Conference Center’, uses small text right-aligned.
5.6 Color Control Strategy
GPT-4o’s understanding of color description is more precise, and you can use the following methods to control color:
- Use precise color names instead of vague descriptions (e.g., “Cobalt Blue” instead of “Blue”)
- Use hexadecimal color codes (e.g., #FF5733) for the most precise color
- Specify complete color schemes (main color, assistant color, emphasized color)
- Describe color relationships (complementary colors, trichromatic colors, analogous colors, etc.)
- Specify color ratio (60-30-10 rule, etc.)
“Design a modern brand identity element set. Uses the following precise color scheme: Main color Cobalt Blue (#0047AB) occupies 60% area, assistant color Coral Red (#FF6B6B) occupies 30% area, emphasized color Mint Green (#98FB98) occupies 10% area. Uses different hue variations of these three colors to create gradient effect. Ensure all color combinations meet WCAG 2.1 AA accessibility standards.”
5.7 Batch Processing Strategy
For projects that require generating a large number of related images, the following strategy can improve efficiency and consistency:
- Create a basic prompt template, only change key elements
- Use the n parameter of the API to generate multiple variants at the same time
- Maintain clear visual style description consistency between batches
- Specify uniform size and ratio for each batch of images
Basic Template:
“Create a 2D advertisement design for a [Product Type]. Place the product in the center, highlighting its advantages. Background uses [Brand Color] gradient, brand logo placed in the bottom right corner. Overall style modern minimalist, suitable for social media distribution.”
Variant 1:
“Create a 2D advertisement design for a Smart Watch. Place the product in the center, highlighting its advantages with Health Monitoring Interface. Background uses Deep Blue to Cyan gradient, brand logo placed in the bottom right corner. Overall style modern minimalist, suitable for social media distribution.”
Variant 2:
“Create a 2D advertisement design for a Wireless Earphone. Place the product in the center, highlighting its advantages with Audio and Noise Reduction Icon. Background uses Deep Blue to Cyan gradient, brand logo placed in the bottom right corner. Overall style modern minimalist, suitable for social media distribution.”
Summary of Advanced Prompting Techniques: Structured Description + Precise Visual Vocabulary + Detail Control + Multi-turn Iteration = Best Generation Result
6. Innovative Use Cases and Case Studies
GPT-4o’s image generation capabilities have opened up new possibilities for various industries. Below are some verified innovative use cases:
6.1 Product Design and Prototype Development
GPT-4o can quickly convert text descriptions into product visual prototypes, significantly accelerating the design iteration process:
- Automated UI/UX Design: Automatically generate interface prototypes by describing feature requirements
- Product Variant Exploration: Quickly generate multiple design options for the same product
- 3D Model Conceptual Diagram: Provide preliminary visual reference for industrial design
Case Study: Mobile Application Design Workflow Optimization
A tech startup used GPT-4o image generation API to automatically convert user stories into UI prototypes, reducing design iteration time by 62%. Designers provided functional descriptions, and the API automatically generated multiple interface options. Designers only needed to make final adjustments and improvements.
6.2 Content Creation and Marketing
Content creators and marketing teams can utilize GPT-4o to generate various visual materials:
- Social Media Images: Automatically generate images matching copy
- Blog Article Images: Generate professional illustrations matching article content
- Advertising Creative: Quickly test different visual style advertising effects
- Content Localization: Generate culture-adapted images for different markets
Case Study: Seasonal Content Update for E-commerce Platform
An e-commerce platform utilized GPT-4o API to automatically generate seasonal marketing images for thousands of products, reducing the original two-week work to 2 days. The system automatically generated images based on product descriptions, season themes, and brand guidelines, significantly improving conversion rates.
6.3 Education and Training
GPT-4o’s application prospects in the education field are vast:
- Concept Visualization: Convert abstract concepts into easily understandable visual representations
- Interactive Learning Materials: Generate related illustrations based on learning content
- Teaching Assistant Tools: Help teachers quickly create visual teaching resources
- Customized Learning Experience: Generate personalized learning materials based on student needs
Case Study: Visual Learning System for Medical Education
A medical school utilized GPT-4o API to develop a visual learning system, allowing students to input medical concepts for instant visual explanations. The system could generate anatomical diagrams, physiological process animations, and disease mechanism diagrams, improving learning efficiency and understanding depth.
6.4 Software Development and Documentation
Development teams can utilize GPT-4o to improve development efficiency:
- System Architecture Diagram: Generate system architecture diagram from text description
- Flowchart and Data Flow Diagram: Visualize complex software processes
- API Documentation Illustration: Add visual illustrations to technical documentation
- User Guide Illustration: Generate step-by-step image instructions
Case Study: Microservice Architecture Visualization Tool
A cloud service provider integrated GPT-4o API to develop an architecture visualization tool. Developers only needed to provide system description, and could obtain detailed architecture diagram. The tool could recognize service relationships and present them visually, improving team collaboration efficiency and system understanding.
6.5 Other Innovative Applications
- Virtual Try-on System: Generate images of people wearing specific clothing
- Indoor Design Visualization: Generate indoor design solutions from text description
- Game Asset Generation: Quickly create game concept art and UI elements
- Medical Image Interpretation: Convert complex medical images into easily understandable diagrams
- Legal Document Visualization: Convert contract terms and legal relationships into charts
7. Comparison with DALL-E 3 and Midjourney
To help you choose the most suitable image generation solution, we conducted a comprehensive comparison of the current market’s mainstream models:
Comparison Dimension | GPT-4o | DALL-E 3 | Midjourney v6 |
---|---|---|---|
Text Understanding | High (Multimodal Understanding) | High | Medium |
Text Rendering Accuracy | Excellent (90%+ Text Accuracy) | Good (70% Text Accuracy) | Average (Often Text Errors) |
Image Quality | High (Especially Technical/Information Graphics) | High | High (Art Effectively Stronger) |
Generation Speed | Fast (2-5 seconds/image) | Medium (5-10 seconds/image) | Slow (30 seconds-2 minutes/image) |
API Integration Difficulty | Low (Standard OpenAI API) | Low (Standard OpenAI API) | High (Limited API Access) |
Customization | High (Multi-turn Dialog Optimization) | Medium | High (Parameter Control) |
Price | Medium | Medium | High |
Most Suitable Scenario | Technical Illustration, UI Design, Information Graphic, Precise Text Rendering | General Image, Product Rendering, Marketing Material | Art Creation, Concept Design, Style Image |
GPT-4o’s biggest advantage is: Text Understanding, Multimodal Interaction, Text Rendering Accuracy, and Generation Speed. If your application needs to precisely follow instructions, present text content, or efficiently batch process, GPT-4o is the best choice.
8. Performance Optimization and Cost Control Strategies
Effective utilization of the GPT-4o image generation API not only requires technical mastery but also requires optimization strategies to control costs and improve performance:
8.1 API Call Optimization Techniques
- Batch Processing Requests: Use the n parameter to generate multiple variants at once instead of multiple calls
- Cache Common Images: Establish local cache for images frequently used
- Reasonable Selection of Resolution: Select the most suitable resolution based on actual needs
- Error Handling and Retry Mechanism: Implement intelligent retry strategy to avoid additional costs
# Optimized batch image generation function
def batch_generate_images(prompts, size="1024x1024", model="gpt-4o"):
"""
Batch generate images and implement intelligent error handling
"""
results = []
for prompt in prompts:
# Generate cache key
cache_key = f"{hash(prompt)}_{size}_{model}"
cache_path = f"image_cache/{cache_key}.png"
# Check cache
if os.path.exists(cache_path):
results.append({"prompt": prompt, "path": cache_path, "cached": True})
continue
# Not cached, try to generate
max_retries = 3
retry_count = 0
while retry_count < max_retries:
try:
image_path = generate_image(prompt, size, 1, model)
# Save to cache
os.makedirs("image_cache", exist_ok=True)
shutil.copy(image_path, cache_path)
results.append({"prompt": prompt, "path": image_path, "cached": False})
break
except Exception as e:
retry_count += 1
if retry_count >= max_retries:
results.append({"prompt": prompt, "error": str(e), "failed": True})
time.sleep(2) # Avoid too frequent requests
return results
8.2 Cost Control Strategy
The following strategies can help you effectively control the cost of GPT-4o image generation:
- Resolution Strategy: First use low resolution for quick test, confirm effect before generating high resolution version
- User Quota Management: Set different generation quotas for different user roles
- Budget Alert: Set API usage threshold, alert when reached
- Use laozhang.ai Platform: Utilize price advantage, save 30% cost
Example: Stepped Resolution Strategy
- Prototype Stage: Use 256×256 resolution for concept verification (lowest cost)
- Iteration Stage: Use 512×512 resolution for detail adjustments (medium cost)
- Final Output: Use 1024×1024 or higher resolution (only for final version)
This strategy can reduce overall costs by about 65%, while ensuring final output quality.
8.3 System Architecture Optimization
For applications that require large-scale image generation, the following architecture optimization is recommended:
- Asynchronous Processing Queue: Use message queue to handle image generation requests
- Result Cache Layer: Establish efficient image cache mechanism
- CDN Integration: Use content delivery network to optimize image loading speed
- On-demand Generation Strategy: Implement delayed loading and on-demand generation mechanism
// Asynchronous image generation architecture example (Node.js)
// Use Redis as cache and message queue
const redis = require('redis');
const { promisify } = require('util');
const client = redis.createClient();
const getAsync = promisify(client.get).bind(client);
const setAsync = promisify(client.set).bind(client);
// Image generation request processor
async function handleImageRequest(req, res) {
const { prompt, size, userId } = req.body;
const cacheKey = `img:${Buffer.from(prompt).toString('base64')}:${size}`;
// Check cache
const cachedResult = await getAsync(cacheKey);
if (cachedResult) {
return res.json({ imageUrl: JSON.parse(cachedResult).imageUrl, cached: true });
}
// Check user quota
const userQuota = await checkUserQuota(userId);
if (!userQuota.hasRemaining) {
return res.status(403).json({ error: 'Image generation quota limit reached' });
}
// Create task ID and add to queue
const taskId = `task:${Date.now()}:${Math.random().toString(36).substring(2, 10)}`;
await client.rpush('image_generation_queue', JSON.stringify({
taskId,
prompt,
size,
userId,
timestamp: Date.now()
}));
// Return task ID, client can use it to query status
res.json({ taskId, status: 'processing' });
}
// Background worker process (run in separate process)
async function imageGenerationWorker() {
while (true) {
// Get task from queue
const task = await client.blpop('image_generation_queue', 0);
const taskData = JSON.parse(task[1]);
try {
// Generate image
const imageResult = await generateImage(taskData.prompt, taskData.size);
// Update user quota
await updateUserQuota(taskData.userId);
// Save result to cache
const cacheKey = `img:${Buffer.from(taskData.prompt).toString('base64')}:${taskData.size}`;
await setAsync(cacheKey, JSON.stringify({ imageUrl: imageResult.url }), 'EX', 86400 * 7); // Cache for 7 days
// Update task status
await setAsync(taskData.taskId, JSON.stringify({
status: 'completed',
imageUrl: imageResult.url
}), 'EX', 3600); // Cache for 1 hour
} catch (error) {
// Handle error
await setAsync(taskData.taskId, JSON.stringify({
status: 'failed',
error: error.message
}), 'EX', 3600);
}
}
}
9. laozhang.ai – The Most Convenient API Access Solution
For Chinese developers and enterprises, laozhang.ai provides the optimal solution for accessing the GPT-4o image generation API:
9.1 Platform Advantage
- Instant Access: No need for overseas credit card, register and use directly
- Stable Connection: Optimized for Chinese network environment, ensure stable access
- Localization Support: Provide Chinese technical documentation and customer service
- Compatible with Native API: Fully compatible with OpenAI native API format, no need to modify existing code
- Price Advantage: Save 20-30% cost compared to direct API calls
- Rich Model Support:In addition to GPT-4o, it also supports multiple AI models
9.2 Onboarding Guide
- Visit https://api.laozhang.ai/register/?aff_code=JnIT to complete registration
- Enter the user console, generate API key
- Set the API endpoint to https://api.laozhang.ai/v1/images/generations according to the standard OpenAI API format (fully compatible, no need to modify existing code)
- Use your API key for authentication
- Start generating high-quality images
New User Exclusive Benefit: Register at laozhang.ai through the link provided in this article and receive an additional $50 worth of free API credits! WeChat consultation: ghj930213
9.3 Enterprise Customization Solution
laozhang.ai also provides customized solutions for enterprise users:
- Exclusive API Channel: Provide independent API channel for large customers, ensure stability
- Bulk Call Discount: Large image generation needs can enjoy more discounts
- Technical Connection Support: Provide one-on-one technical support, assist system integration
- Custom Development Service: Can provide customized development based on enterprise needs
Enterprise consultation contact: ghj930213
10. Frequently Asked Questions
What’s the difference between GPT-4o image generation API and DALL-E 3?
GPT-4o image generation is based on a more advanced multimodal model, compared to DALL-E 3, it has stronger text understanding, higher text rendering accuracy, and faster generation speed. It’s especially suitable for scenarios requiring precise expression of complex concepts, such as technical illustrations, information graphics, etc.
How to improve the quality of GPT-4o generated images?
The key to improving image quality is to provide structured, detailed prompts. Follow the prompting techniques provided in Section 5 of this article, especially including theme description, specific details, visual style, composition guidelines, and technical parameters. Multi-turn dialog optimization is also an effective method to improve quality.
What are the usage restrictions of the GPT-4o image generation API?
The main restrictions include content policy (prohibiting generation of violent, pornographic, etc. illegal content), rate limit (request number limit per minute), and resolution limit (maximum support 4096×4096).
Who owns the generated images?
The usage rights of images generated through the GPT-4o API belong to the creator. OpenAI’s usage terms allow commercial use, but do not guarantee the uniqueness of generated content. It’s recommended to perform appropriate uniqueness check in commercial projects.
How to handle API request failure or timeout?
It’s recommended to implement intelligent retry mechanism, use exponential backoff strategy to handle temporary errors, and set reasonable timeout threshold. For batch processing, you can implement queue system to ensure task completion, as shown in Section 8 architecture example.
What’s the difference between laozhang.ai and directly using OpenAI API?
laozhang.ai provides interface fully compatible with OpenAI API, but optimized for Chinese users: No need for overseas payment method, More stable network connection, Chinese support service, More favorable price and More relaxed usage restrictions. Code is fully compatible, only need to modify API endpoint.
What formats and sizes does GPT-4o generate images?
Supports PNG, JPEG, and WEBP format outputs, Resolution options include 256×256 to 4096×4096 multiple sizes. You can specify the required format and size through API parameters, see Section 3 Technical Specifications for details.
How to handle large-scale image generation without exceeding API limit?
Implement asynchronous processing queue, distributed processing, request throttling, and intelligent retry mechanism can effectively handle large-scale needs. At the same time, laozhang.ai provides higher API call limit and exclusive channel for enterprise users, suitable for large-scale production environment.
Summary
GPT-4o image generation API represents a major leap in AI image generation technology, particularly in text understanding, precise rendering, and generation speed. Through the API access method, code example, prompting technique, and optimization strategy provided in this article, developers can fully unleash the potential of GPT-4o image generation, creating high-quality, precise visual content that meets specific needs.
For Chinese developers, laozhang.ai provides the most convenient and economical access solution, without complicated application process, to enjoy GPT-4o’s powerful image generation capabilities. Register immediately, get $50 worth of free experience credit, and start a new era of AI image generation!
👉 Register laozhang.ai, Get GPT-4o Image Generation API Access
👉 Any questions, please add customer service WeChat: ghj930213